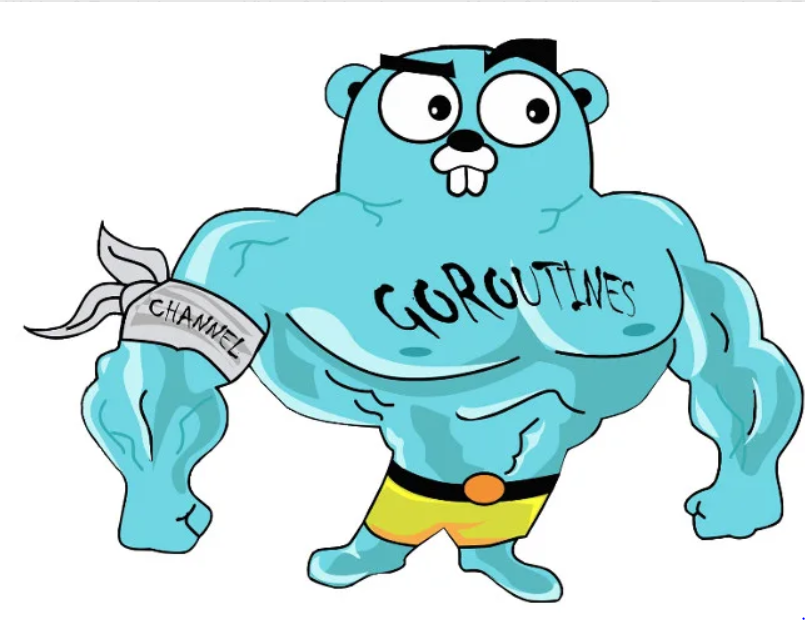
Are you a developer in the making? Does the thought of learning a new trending language excite you? Stay with me. Because that was me around a year back when I first heard about ‘Golang’.
There’s a difference between ‘learning a language’ and ‘knowing a language’. I believe that to really get comfortable with a language you need to focus more on the very basics of it rather than the fancy stuff. And in order to do that, one needs a certain amount of excitement about the concepts being learned and to realize how this new horse in the race overcomes the challenges faced by other languages, and that’s exactly what I intend to provide you with, in this article concentrating specifically on ‘Golang’.
What is Go?
Go is an open-source programming language that makes it easy to build simple, reliable, and efficient software. It was designed at Google by Robert Griesemer, Rob Pike, and Ken Thompson, and the world was first introduced to Go in 2009.

The Goods
There is a fair share of reasons why I absolutely loved working with Go. Some of which I have highlighted here:
1. Ease of Use
Go provides remarkable clarity and very less ambiguity with its syntax.
Consider a simple scenario where you have an array or maybe a list of data items and you want to simply traverse that data. Anyone who is even sparsely familiar with coding would know that the obvious answer is ‘looping’. Languages like Java, C++, and Javascript provide you with a variety of loops accomplishing different purposes, namely – for, while, do/while, for/in, for/of, range, etc.
Go also provides you with some looping mechanisms, namely – for …… That’s it! And that doesn’t mean you miss out on any of the above-mentioned functionalities of looping. All you have to do is use this ‘for’ loop as per your requirement. Below is a use case for reference:-
package main
import "fmt"
//'While Loop'
func main() {
sum := 1
for sum < 1000 {
sum += sum
}
fmt.Println(sum)
}
// 'For Loop'
func main() {
sum := 0
for i:=0; i < 10; i++ {
sum += i
}
fmt.Println(sum)
}
You might have also noticed some major syntax changes like the absence of semicolons and parentheses. And not only for loop, features like – the ability to provide a small initial statement to be executed before ‘if’ conditions, no need for ‘breaks’, and running selective cases when using ‘switch-cases’ are some other examples. Many more can be found at A tour of Go.
I would highly recommend taking a walk through this and trying to compare the basic features of Go with the programming language of your choice.
2. Multithreading/Parallel Programming
Honestly speaking, now this is something I was afraid of in college, running several threads or logics parallelly, sounds complex right? Well, it is, I mean so much can go wrong, race situations, deadlocking, cache issues, garbage memory allocation, improper sync, and whatnot. But I was more than delighted when I wrote my first code with multiple threads and it seemed like anything was possible, but what does it have to do with ‘Go’? Well, it was also my very first program in ‘Go’. And that was when I realized that Go made multithreading look so easy! Everything is good with examples, so let me take a small one.
Let’s assume there is a restaurant, and a normal transaction here goes like this:
- The waiter takes the order from the customer and takes it to the kitchen.
- The kitchen makes food and the waiter takes it to the customer.
- The customer eats and the waiter takes back empty plates to the kitchen.
- The waiter delivers the bill to the customer and the customer pays.
- The waiter takes the money to the kitchen and the kitchen creates a receipt
- The waiter delivers the receipt to the customer.
And our transaction is complete. Simple isn’t it? Now let’s code it.
package main
import (
"fmt"
)
func kitchen(waiter1 chan string, waiter2 chan string) {
var to_customer string
for {
from_customer:=<-waiter1
switch fmt.Println(from_customer,"delivered to the Kitchen");from_customer{
case "Order":
to_customer="Food"
case "Empty Plates":
to_customer="Bill"
case "Money":
to_customer="Receipt"
default:
fmt.Println("Incorrect item recieved from customer.")
}
waiter2<-to_customer
}
}
func main() {
var from_kitchen string
var to_kitchen string
//Two waiters, one for providing items from customer to kitchen and another
//for vice-versa
waiter1:= make(chan string, 1)
waiter2:= make(chan string, 1)
//Calling function kitchen in a seperate sub-routine
go kitchen(waiter1,waiter2)
waiter1<-"Order"
for from_kitchen!="Receipt"{
from_kitchen=<-waiter2
switch fmt.Println(from_kitchen,"delivered to the Customer");from_kitchen{
case "Food":
to_kitchen="Empty Plates"
case "Bill":
to_kitchen="Money"
case "Receipt":
to_kitchen=""
default:
fmt.Println("Incorrect item recieved from kitchen.")
}
waiter1<-to_kitchen
}
fmt.Println("Transaction complete.")
}
This is a very simple code I wrote to illustrate the given example. Here, channels ‘waiter1’ and ‘waiter2’ are used to communicate between the main thread and a sub-thread initiated by using a simple keyword ‘go’. These threads are known as ‘Routines’ in ‘Go’ terminology and here represent the entities ‘Customer’ and ‘Kitchen’.
Did you notice how easy it was to visualize the scenario just by looking at the code?
To better understand the functioning of routines you can just copy-paste this code and play with it a bit here: Go Playground.
Also, channels are the most basic tool to drive routines, there are some more tools like Waitgroups, Ranges, Mutexes, etc. that you should definitely know about if you are using routines. You can follow up on these here: Go By Example.
3. Very, Very Good Documentation
I can’t emphasize it enough, but Go libraries do contain really impressively detailed documentation. This might not sound like a big pro but believe it makes a huge difference. When I was getting myself started working with Go, I rarely remember hitting ‘stackoverflow.com’ or other discussion forums when I was stuck. All I had to do was read about the library or the package in Go’s official documentation, and most of the time it would be enough to move forward with my work. Good documentation not only helps newcomers to get a grasp quickly but also saves a significant amount of developer time.
You will most probably find everything you need here: Go Docs.
So these were some things that I really liked about ‘Go’. If you are following me till here, I’m pretty sure you must have developed a liking for the language, but the next part of the article is as important.
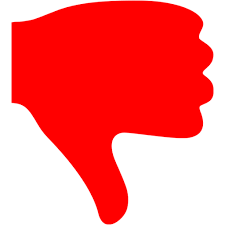
The Bads
As the saying goes ‘No language is perfect’ and ‘Go’ is no exception. Some of the key drawbacks are:
1. Maturing fast but still young
When compared to ancient languages like Java, Go is still quite young. The library support for these established languages and the engagement of enthusiasts is huge, Go is found lacking this.
2. RAM eater
Go is not based upon a Virtual Machine in order to provide ease of use, but this, in turn, increases the Go file sizes. And if you are hoping to build a large-scale complex application, then you would have to be ready with some spare RAM. Although Google has been slowly improving efficiency lately.
3. Security Issues
Go has always been inclined towards speed and ease, which makes it lack in other aspects. And one of these aspects is runtime vulnerability.
Closing Thoughts
‘Go’ or ‘Golang’ is an easy-to-learn, and easy-to-use language. It focuses on speed and has very good documentation, which makes it idle for fast-paced development. Apart from these, it also enables the developer to maintain a clean and easy-to-understand codebase. It does outperform some other languages in some aspects. Still ‘Go’ does not prove to be the first choice when it comes to large-scale applications due to the above-mentioned reasons and still has some way to go before taking its place among other prominent players.